C++ is a powerful and versatile programming language that can be used to create applications for various platforms and purposes. One of the common tasks that C++ developers may encounter is how to communicate with a web server using HTTP methods, such as GET and POST. HTTP stands for Hypertext Transfer Protocol, and it is the standard way of exchanging data between a client and a server on the web. GET and POST are two of the most widely used HTTP methods, and they have different functions and characteristics.
To send and receive data with GET and POST to a web server, you need to understand how the HTTP protocol works. HTTP is a standard way of communicating between a client (such as a web browser) and a server (such as a web server) over the internet. HTTP uses different methods to specify what kind of action the client wants to perform on the server. The most common methods are GET and POST.
GET is used to request data from the server, such as a web page, an image, or a file. The data is usually encoded in the URL of the request, after a question mark (?). For example, if you want to search for "php", you can use the following URL:
https://learn-tech-tips.blogspot.com/search?q=php
The part after the question mark is called the query string, and it contains one or more name-value pairs separated by ampersands (&). In this case, the name is "q" and the value is "php". The server can use this information to process the request and return the appropriate response.
POST is used to send data to the server, such as a form submission, a file upload, or an API call. The data is usually encoded in the body of the request, not in the URL. For example, if you want to create a new account on a website, you may need to fill out a form with your username, password, email, etc. and click a submit button. The browser will then send a POST request to the server with the form data in the body.
Also, additional steps are needed to add the request body data. With cURL, you can pass a string or other data object to the request handle to attach as the POST body content. For structured data, this is often JSON encoded. With Boost.Asio, you create a request object, fill out the body, and pass this to the client for sending.
POST /form.php HTTP/1.1
Host: www.example.com
Content-Type: application/x-www-form-urlencoded
Content-Length: 13
name=WebzoneTechTipsZidane&age=28
The first line specifies the method, the path, and the protocol version. The next lines are the headers that provide additional information about the request, such as the host name, the content type, and the content length. The last line is a blank line that separates the headers from the body, which contains the data to be sent to the server. POST requests are more flexible and secure than GET requests, as they can send large amounts of data and hide them from the browser's address bar. POST requests are usually used for sending data that changes the state of the server, such as creating or updating records in a database.
When working with web APIs, C++ can format requests and parse responses in JSON or XML based on API specs. Popular JSON libraries like nlohmann/json can serialize C++ objects to JSON for the request body and deserialize JSON responses.
Proper error handling is important when working with network requests. Checking return codes for potential timeouts, connection issues or HTTP status codes other than 200 OK should be handled gracefully.
Authentication is also common, requiring adding HTTP headers or request body data for credentials like API keys, OAuth tokens or basic auth. Session handling may also be necessary to retain and send cookies or other metadata.
Overall, C++ provides low level control for interfacing with web servers and APIs. With its performance and direct memory access, it can efficiently send, receive and process web data. Using libraries handles much of the connection and protocol details.
Below is demo source code detail use GET POST with C++ and PHP programing language for above information
Full source code
You can download my source code from here
Lib
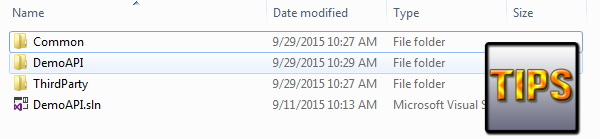
- Common contains Include folder
-> RegExp.h, StringProcess.h, WinHttpClient.h
- ThirdParty contains ATLRegExp folder -> atlrx.h
Path
https://localhost/getpostdemo.php?name=zidane&age=18
PHP code:
<?php
$yourname = isset($_POST['name']) ? $_POST['name'] : 'no name';
$yourage = isset($_POST['age']) ? $_POST['age'] : 'no age';
echo $yourname . " - " . $yourage;
?>
Result:
Zidane - 18
C++
Add Lib to Projects- Right click to projects -> Properties -> Additional Include Directories -> ..\Common\Include
Source code using GET method
CString CGetPostDemo::SendGetData(void)
{
// Set URL.
WinHttpClient client(L"https://localhost/checkdb.php?name=zidane&age=12");
// Send https request, a GET request by default.
client.SendHttpRequest();
// The response header.
wstring httpResponseHeader = client.GetResponseHeader();
// The response content.
wstring httpResponseContent = client.GetResponseContent();
CString szMsg = L"";
szMsg.Format(L"%s", httpResponseContent);
return szMsg;
}
Explore My Other Channel for More Cool and Valuable Insights
👉 Youtube Learn Tech Tips👉 Tiktok
👉 Facebook:CString
CGetPostDemo
::SendPostData(void) { WinHttpClient client(L"https://localhost/checkdb.php"); // Set post data. string data = "name=zidane&age=12"; client.SetAdditionalDataToSend((BYTE *)data.c_str(), data.size()); // Set request headers. wchar_t szSize[50] = L""; swprintf_s(szSize, L"%d", data.size()); wstring headers = L"Content-Length: "; headers += szSize; headers += L"\r\nContent-Type: application/x-www-form-urlencoded\r\n"; client.SetAdditionalRequestHeaders(headers); // Send https post request. client.SendHttpRequest(L"POST"); wstring httpResponseHeader = client.GetResponseHeader(); wstring httpResponseContent = client.GetResponseContent(); CString szMsg = L""; szMsg.Format(L"%s", httpResponseContent); return szMsg; }
CGetPostDemo.h
#include <string> #include <iostream> using namespace std; class CGetPostDemo { public:
CGetPostDemo
(void); ~
CGetPostDemo
(void); static CString SendGetData(void); static CString SendPostData(void); };
CGetPostDemo.cpp
// huuvi168@gmail.com (zidane)
#include "stdafx.h"
#include "GetPostDemo.h"
#include "WinHttpClient.h"
CGetPostDemo::CGetPostDemo(void)
{
}
CGetPostDemo::~CGetPostDemo(void)
{
}
CString CGetPostDemo::SendPostData(void)
{
WinHttpClient client(L"https://localhost/getpostdemo.php");
// Set post data.
string data = "name=zidane&age=12";
client.SetAdditionalDataToSend((BYTE *)data.c_str(), data.size());
// Set request headers.
wchar_t szSize[50] = L"";
swprintf_s(szSize, L"%d", data.size());
wstring headers = L"Content-Length: ";
headers += szSize;
headers += L"\r\nContent-Type: application/x-www-form-urlencoded\r\n";
client.SetAdditionalRequestHeaders(headers);
// Send https post request.
client.SendHttpRequest(L"POST");
wstring httpResponseHeader = client.GetResponseHeader();
wstring httpResponseContent = client.GetResponseContent();
CString szMsg = L"";
szMsg.Format(L"%s", httpResponseContent);
return szMsg;
}
CString CGetPostDemo::SendGetData(void)
{
// Set URL.
WinHttpClient client(L"https://localhost/getpostdemo.php?name=zidane&age=12");
// Send https request, a GET request by default.
client.SendHttpRequest();
// The response header.
wstring httpResponseHeader = client.GetResponseHeader();
// The response content.
wstring httpResponseContent = client.GetResponseContent();
CString szMsg = L"";
szMsg.Format(L"%s", httpResponseContent);
return szMsg;
}
#C++,
#HTTP,
#GET,
#POST,
#WebServer,
#libcurl,
#BoostBeast,
#POCO,
#QtNetwork,
#NetworkProgramming
Are you interested in topic How to send and receive data with GET, POST to Web Server from Webzone Tech Tips? If you have any thoughts or questions, please share them in the comment section below. I would love to hear from you and chat about it
Webzone Tech Tips Zidane